Python Programming for Kids and Teenagers: Beginner to Advanced
- Duration: 6 months
- Level Beginner
- Age: 12yrs +
About This Course
This course is designed for young learners aged 12 and above who are eager to dive into the programming world using Python. Starting with the basics and advancing to more complex concepts, this course will equip students with the skills they need to create their own programs, games, and applications. Through interactive lessons, hands-on projects, and creative challenges, students will build a strong foundation in Python and develop problem-solving skills that will serve them in any future coding endeavors.
Target Audience
- Age Group: 12 – 17 years (and above)
- Prerequisites: No prior programming experience is required. A willingness to learn and explore is all you need!
Support and Community
- Live Sessions: Interactive classes twice a week where students can ask questions and engage directly with instructors.
- Pre-recorded Videos: Comprehensive weekly lessons that students can watch at their own pace.
- Community Forum: An online platform where students can interact, share ideas, collaborate on projects, and help each other solve problems.
- Tutor Support:
- Office Hours: Dedicated time slots where tutors are available for one-on-one assistance.
- Email Support: Students can reach out to instructors for help and clarification.
- Feedback: Regular constructive feedback on assignments and projects to aid improvement.
- Parental Updates: Periodic reports on student progress to keep parents informed and engaged.
Evaluation Method
- Mini Projects: Students will complete small projects at the end of each lesson to reinforce learned concepts.
- Quizzes: Short Quizzes at the end of each module to revise and evaluate learned concepts and theories.
- Final Projects: Students will develop a complex project of their choice, incorporating various elements learned throughout the course.
Course Objectives
By the end of this course, students will be able to:
- Understand the fundamentals of Python programming, including syntax, variables, data types, and control flow.
- Apply programming logic using conditional statements and loops to solve problems.
- Write reusable and modular code using functions and libraries.
- Work with data structures such as lists, tuples, dictionaries, and sets to manage and manipulate data.
- Create simple graphical applications and games using PyGame.
- Debug and troubleshoot code effectively.
- Develop their own projects, applying the skills learned throughout the course.
- Develop and improve on computational and creative thinking skills.
- Practice effective leadership, team, and collaboration skills that are applicable across various works of life.
- Develop interest and brilliance in mathematics, which in turn improves their intelligence quotient (IQ).
Curriculum
Lesson 1: Getting Started with Python
- What is Python? Introduction to programming.
- Setting up the development environment (IDLE, Thonny).
- Writing and running your first Python program (print("Hello, World!")).
- Understanding basic syntax and structure.
- Activity: Write a simple Python program to print your name and a short greeting.
Lesson 2: Variables and Data Types
- What are variables? How to declare and use them.
- Introduction to basic data types: integers, floats, strings.
- Performing simple arithmetic operations.
- Understanding the print and input functions.
Activity: Create a program that asks for the user's name and age, then prints a personalized message.
Lesson 3: Conditional Statements
- Introduction to if, elif, and else statements.
- Using comparison operators (==, !=, >, <, etc.).
- Combining conditions with logical operators (and, or, not).
Activity: Build a simple quiz program that gives feedback based on user answers.
Lesson 4: Loops
- Introduction to loops: for loops and while loops.
- Understanding loop control statements: break, continue.
- Creating simple patterns and sequences using loops.
Activity: Create a program that prints the first 10 multiples of a number provided by the user.
Lesson 5: Creating and Using Functions
- What are functions? Benefits of using functions.
- Defining and calling functions.
- Passing arguments and using return values.
- Understanding scope: local and global variables.
Activity: Write a function that calculates and returns the area of a rectangle given its length and width.
Lesson 6: Introduction to Modules and Libraries
- What are modules? How to import and use them.
- Exploring Python's standard libraries (e.g., math, random).
- Creating and using your own modules.
Activity: Use the random module to create a simple dice-rolling game.
Lesson 7: Working with Lists
- Introduction to lists: creating, accessing, and modifying elements.
- List methods: append, remove, pop, sort, reverse, etc.
- Iterating over lists using loops.
Activity: Create a program that manages a simple to-do list.
Lesson 8: Tuples and List Comprehensions
- Understanding tuples: creating and using immutable sequences.
- Differences between lists and tuples.
- Introduction to list comprehensions for concise code.
Activity: Create a program that stores and prints a list of favorite movies or games using tuples and list comprehensions.
Lesson 9: String Manipulation
- Understanding strings and their properties.
- String methods: lower, upper, replace, find, split, join.
- String formatting using f-strings and the format method.
Activity: Create a program that takes a sentence as input and outputs the sentence in reverse order.
Lesson 10: Introduction to File Handling
- Opening and closing files using with statements.
- Reading from and writing to files (read, write, append modes).
- Handling errors when working with files.
Activity: Modify the to-do list program to save tasks to a file and load them on startup.
Lesson 11: Understanding Dictionaries
- Introduction to dictionaries: key-value pairs.
- Accessing, adding, and modifying dictionary elements.
- Dictionary methods: keys, values, items, get, pop.
Activity: Create a program that stores contact information (name, phone number, email) using a dictionary.
Lesson 12: Working with Sets
- Introduction to sets: unique collections.
- Basic set operations: union, intersection, difference.
- Using sets to remove duplicates from a list.
Activity: Create a program that compares two sets of favorite items (e.g., favorite books) and finds common items.
Lesson 13: Handling Errors and Exceptions
- Understanding common errors: syntax errors, runtime errors.
- Using try, except, finally blocks to handle exceptions.
- Creating custom error messages for user input validation.
Activity: Create a program that asks for a number and handles cases where the user inputs invalid data.
Lesson 14: Debugging Techniques
- Using print statements to trace errors.
- Introduction to debugging tools in the IDE.
- Identifying and fixing logical errors in code.
Activity: Provide a buggy code snippet for students to debug and fix.
Lesson 15: Classes and Objects
- What is OOP? Understanding classes and objects.
- Defining a class and creating objects.
- Using methods and attributes.
Activity: Create a class for a simple game character with attributes like name, health, and actions like attack, heal.
Lesson 16: Inheritance and Polymorphism
- Introduction to inheritance: creating subclasses.
- Understanding polymorphism and method overriding.
- Creating hierarchies of related classes.
Activity: Extend the game character class to create different types of characters (e.g., warrior, mage) with unique abilities.
Lesson 17: Setting Up Pygame
- Installing Pygame and setting up the environment.
- Understanding basic Pygame concepts: surfaces, events, main loop.
- Drawing shapes and displaying images.
Activity: Create a simple Pygame window with a moving object.
Lesson 18: Creating a Simple Game
- Handling user input (keyboard and mouse).
- Adding collision detection and game mechanics.
- Keeping score and adding game over conditions.
Activity: Develop a simple game (e.g., a basic platformer or a shooting game) using Pygame.
Lesson 19: Final Project Planning and Development
- Choosing a final project based on student interest.
- Planning the project: breaking it down into tasks.
- Starting the coding process with guidance from the instructor.
Activity: Begin working on a final project, such as a text-based adventure game, a drawing app, or a simple calculator with a history feature.
Lesson 20: Final Project Presentation and Course Review
- Completing and polishing the final project.
- Presenting the project to the class.
- Reviewing key concepts learned throughout the course.
Activity: Students present their projects, demonstrating the features and discussing what they learned. The instructor provides feedback and encouragement.
Interactive Quiz Game
- Description: Create a multiple-choice quiz game that asks the user a series of questions and tracks their score. Include features like different categories of questions, a timer for each question, and feedback based on the user's answers.
- Skills Used: Loops, conditionals, functions, file handling (for storing questions), and possibly simple graphics using tkinter.
Simple Drawing Application
- Description: Develop a basic drawing app where users can draw shapes (lines, circles, rectangles) and choose colors. Implement options to save the drawings and clear the canvas.
- Skills Used: GUI programming with tkinter, event handling, and basic file handling.
Text-Based Adventure Game
- Description: Build a text-based adventure game where players navigate through different scenarios by making choices. The game can include inventory management, multiple endings, and puzzles.
- Skills Used: Control flow, functions, dictionaries (for managing inventory), and file handling.
Personal Diary Application
- Description: Create a personal diary or journal app where users can write and save their entries. Implement features like viewing past entries, searching by date, and password protection.
- Skills Used: File handling, string manipulation, basic encryption (for passwords), and GUI with tkinter.
Simple Budget Tracker
- Description: Develop a budget tracking application that allows users to input their income and expenses. The app should display a summary of spending categories and help users manage their budget.
- Skills Used: Data structures (lists, dictionaries), file handling, loops, and conditional statements.
Memory Matching Game
- Description: Create a simple memory matching game where players flip cards to find matching pairs. The game should include a timer, a scoring system, and a way to reset the game.
- Skills Used: GUI programming with tkinter, loops, and event handling.
Weather App
- Description: Develop a weather application that fetches and displays the current weather for a given location using an API (e.g., OpenWeatherMap). Include features like temperature, humidity, and weather conditions.
- Skills Used: Working with APIs, data parsing (JSON), and GUI programming.
Rock, Paper, Scissors Game
- Description: Build a Rock, Paper, Scissors game where users play against the computer. Add features like score tracking, best of three matches, and difficulty levels.
- Skills Used: Control flow, random module, functions, and possibly a simple GUI with tkinter.
Virtual Pet
- Description: Create a virtual pet game where players can feed, play with, and take care of a digital pet. The pet should have attributes like hunger, happiness, and energy, which change based on player interactions.
- Skills Used: Classes and objects, loops, conditional statements, and file handling (for saving pet status).
Calculator with History
- Description: Develop a calculator application that performs basic arithmetic operations and keeps a history of calculations. Users should be able to view, clear, and save the history to a file.
- Skills Used: Functions, file handling, and possibly a simple GUI with tkinter.
Please note the following:
- As deemed necessary, the curriculum outlined above may undergo further modifications, including additions or deletions. Regardless of the situation, these changes are intended to better fulfil the objectives of the program.
- The extra-curricular activities/sessions (e.g. leadership, soft skills, mathematics clinic sessions, etc.) are embedded across the learning path. Every session will be announced ahead.
- These projects not only help students apply what they’ve learned but also allow them to express their creativity and build a portfolio of work that they can be proud of. We shall also provide the necessary project guidelines to each student and as well monitor their progress and help them with their challenges in appropriate and essential ways.
Certification
Participants who successfully complete the Python Programming course will be awarded a certificate of achievement. This certificate formally recognizes the skills and knowledge acquired. To earn this certificate, participants must complete all course modules and tasks, achieve the passing mark on quizzes, and submit an end-of-course project that passes assessment and approval.
Requirements
Computer/Laptop:
- Operating System: Windows 10 or higher, macOS 10.13 (High Sierra) or higher, or any recent Linux distribution.
- Processor: Intel Core i3 or equivalent.
- Memory (RAM): 4 GB minimum (8 GB recommended).
- Storage: At least 10 GB of free disk space.
- Display: 13-inch screen with a resolution of 1366 x 768 pixels or higher.
- Internet Connectivity: Reliable broadband connection for downloading resources and attending live sessions.
Tablet (Optional):
- Operating System: iOS 12 or higher, Android 8.0 or higher.
- Screen Size: 10 inches or higher for better readability.
- Storage: 32 GB minimum, with free space for software installation
Python Installation:
- Python Version: Python 3.8 or higher.
- Integrated Development Environment (IDE):
- Recommended: Thonny (Beginner-friendly IDE for Python).
- Alternatives: PyCharm (Community Edition), Visual Studio Code, or Jupyter Notebook.
- Installation Instructions: Detailed instructions will be provided in the course materials.
Additional Software:
- Text Editor: Any text editor like Notepad++ (Windows), TextEdit (macOS), or Gedit (Linux).
- Web Browser: Latest version of Chrome, Firefox, Safari, or Edge.
- PDF Reader: Adobe Acrobat Reader or any other PDF reading software to access course materials.
- External Mouse: Recommended for easier navigation and coding, especially for younger students.
- Headset with Microphone: Useful for participating in live sessions and discussions.
- Learning Management System (LMS): The course will be hosted on the Emostel Academy’s LMS platform. Students will need a registered account to access course content, assignments, and live sessions.
- Video Conferencing Software:
- Zoom/Google Meet: For live classes, discussions, and Q&A sessions. Students should have the software installed and be familiar with joining meetings.
- Zoom/Google Meet: For live classes, discussions, and Q&A sessions. Students should have the software installed and be familiar with joining meetings.
- Online Code Editors (Optional):
- Repl.it: A cloud-based coding environment for Python that requires no installation.
- Google Colab: Another cloud-based Python environment that allows code execution directly from the browser.
- Online Python (Beta): A website which offers an online-integrated Python development environment (IDE), allowing users to build, run, and share Python code directly in their web browser
FAQs
Our extracurricular activities encompass a variety of training workshops aimed at providing students with an advantage beyond the standard course curriculum. Participants in these workshops will develop leadership abilities and essential soft skills, which are crucial for success in their immediate surroundings, including home, school environments, and social settings.
These workshops are typically scheduled for weekends, with dates distributed throughout the program's duration and announced according to schedule. Similar to regular live classroom sessions, these workshops are recorded for later reference and participants are allowed to interact freely with their peers and facilitators.
While the course includes live classes twice a week, students have daily access to the learning portal and learners' community. Here, they can reach out to our readily available online tutors via email for support. Additionally, they can connect with tutors through the learners' community or use the designated spaces in the learning portal to post support requests.
On specific days of the week, we provide general tutoring for students on subjects and topics relevant to their classes/grades. For this, participants are organized into communities based on their class levels.
The details we gather through the application form help us in making important decisions as regards this matter as well as other program operations and activities.
- Click on "Join Course" or use the "Enroll in this course" button below.
- Fill out the application form and submit it. An email will be sent to you to confirm the application and provide you with your login details, payment options, and other essential information.
- Make payment for the course via the available channels provided.
- Once your payment is received, you will be granted an access to the chosen course and an email will be sent to you containing the course access details and any other relevant information.
If you cannot access the course within 24hrs, please contact us via sales@emostelacademy.org or (via SMS, WhatsApp, or Phone Call) at +2349077471007, +2348141897754.
You have the option to make a single payment of ₦100,000 for the course or opt for a monthly payment of ₦20,000 for 6 months.
With the monthly plan, access is automatically revoked every 30 days from the date of the last payment unless the subscription is renewed.
For requests/orders, transaction issues, or further assistance regarding your payment, please contact us via sales@emostelacademy.org or (via SMS, WhatsApp, or Phone Call) at +2349077471007, +2348141897754
Each course you enroll for, appears in your personal learner's portal and can be found under "My Courses" tab on your dashboard.
Your login information comes with your course access email.
We have pre-recorded sessions (released weekly) and are supported by live sessions (which are held twice a week).
Every live session is recorded and then posted to the learners' portal. This would be useful for anyone who missed the class to access them later. The recording also aids in further learning and revision.
Apart from the recorded and live sessions, we add our learners to communities based on their courses, allowing them to interact with course instructors, community moderators, and fellow learners, who are always available to help with any issues relating to the course. Learners may also directly contact their instructors via email and other communication channels provided.
For a more personalized experience, you may subscribe to our Private Training Services. This is however optional, it comes at an extra cost and is based on request.
This course is designed to be covered within three months. However, we cater to children with diverse learning abilities and preferences, tailored to their unique personalities. Therefore, we provide an extended duration that allows for comprehensive learning and practical application of the material.
A one-time payment ensures they incur no additional costs until the course access expires. In contrast, those opting for monthly payments must continue to pay for ongoing access and support until their needs are met.
Yet, we commit to giving each student's needs the highest priority to ensure they achieve excellence in the most efficient time frame possible.
Access to the course will expire six months after release, but technical support for participants will be available for 12 months.
This 12-month technical support applies solely to the chosen iCode course. Tutoring for school assignments and extracurricular activities is available only during the six-month access period.
We encourage you to sign up for more courses after completion to expand your knowledge and retain the advantages and support associated with each iCode course. Alternatively, you can opt for monthly payments or a one-time six-month access fee to continue access to perks and benefiting from our tutoring support for your school and homework.
For further inquiries, please contact us via our helplines or at study@emoselacademy.org
Please visit https://emostelacademy.org/faq for more frequently asked questions regarding our mode of class delivery and other relevant information, or contact our support team via email: support@emostelacademy.org
If you wish to learn more about us (who we are, where we are, what we do, etc.), please Click Here.
Course Features
- Registration Starts Oct. 20, 2024
- Language - English
- Personal Student/Learner's Portal
- Pre-recorded Video released weekly. Live sessions hold twice a week
- Lesson can be access on all devices but practiced on desktop and tablet
- Expert Tutor Support for Math Problems and School/Homework
- Leadership Training and Soft Skills Development
- Tutor and Community Support
- 6 months access to course materials but lifetime access to community
- Certificate of Completion
- 10 Modules, 10 Quizzes, 20 Lessons, 63 Topics, 13 Mini Tasks, 6 In-Course Projects, 10 Post-Course Projects
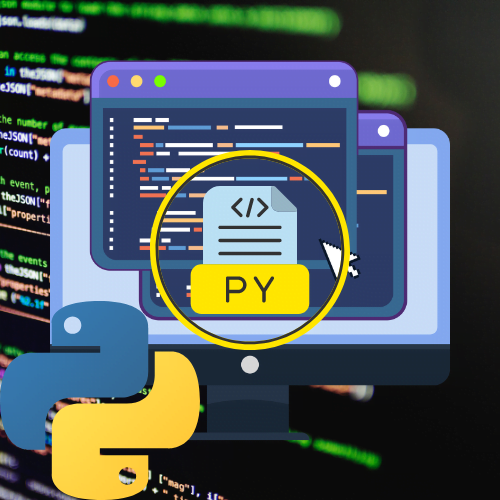